Working with Advanced Topics in SQL.(16)
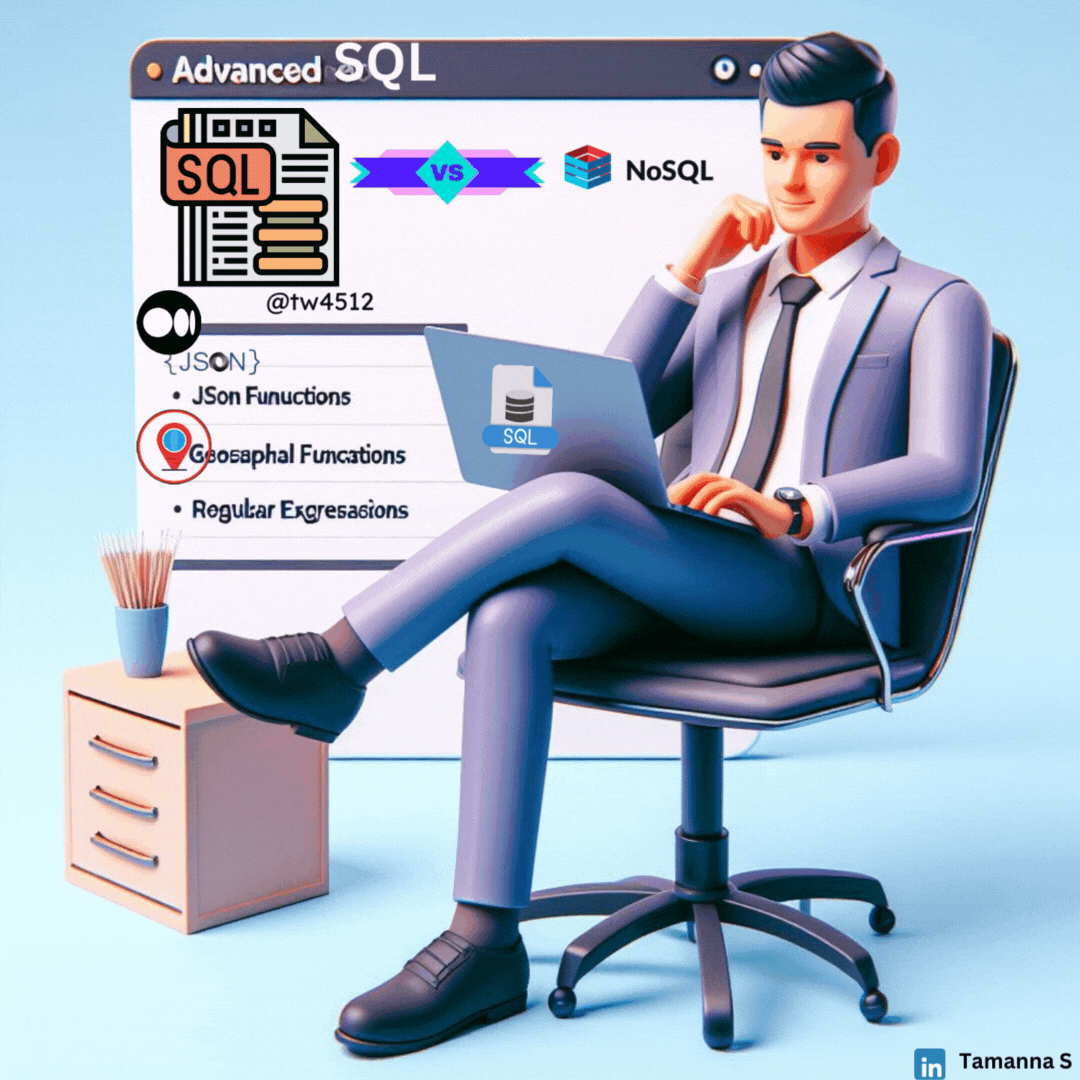
SQL is a powerful and versatile language that can be used to manipulate and analyze data from relational databases. However, SQL is not limited to basic operations such as selecting, inserting, updating, and deleting data. There are many advanced features and functions that can help you perform complex tasks and queries with SQL. In this post, we will explore some of these advanced topics in SQL, such as JSON functions, geospatial functions, regular expressions, and NoSQL vs. SQL. We will also provide examples and references for further learning. This post is part of the SQL Learning Series, a comprehensive guide to learn SQL from basic to advanced level.
JSON Functions:
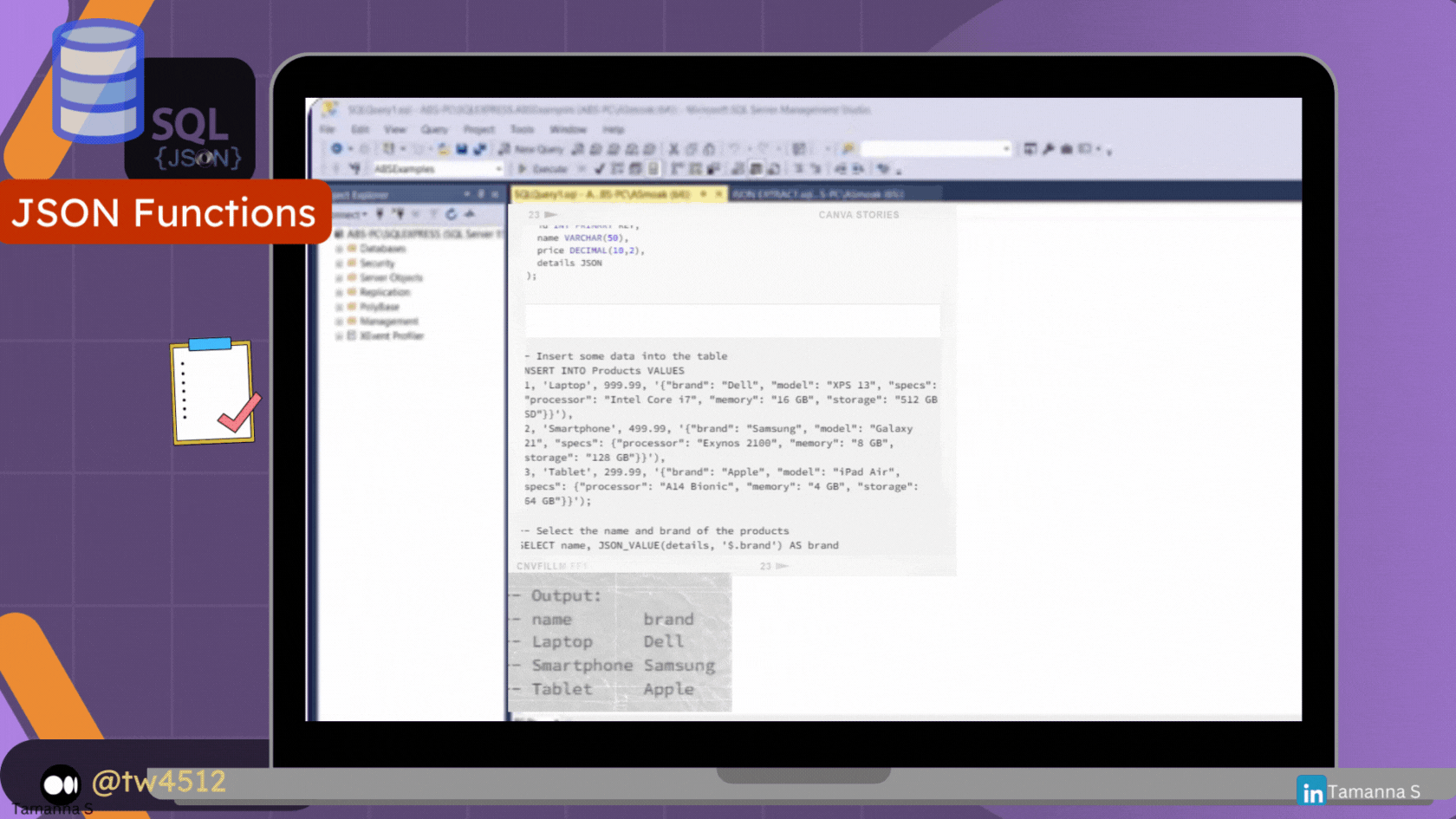
JSON (JavaScript Object Notation) is a popular data format that is widely used for exchanging and storing data on the web. JSON data can be represented as a collection of key-value pairs, where the keys are strings and the values can be strings, numbers, booleans, arrays, or objects. JSON data can be easily parsed and manipulated by various programming languages and frameworks.
SQL also supports JSON data, and provides several functions to work with JSON data. For example, you can use the JSON_VALUE
function to extract a scalar value from a JSON object, or the JSON_QUERY
function to extract an array or an object from a JSON object. You can also use the JSON_MODIFY
function to update a value in a JSON object, or the JSON_CONTAINS
function to check if a JSON object contains a specific value. Here is an example of using JSON functions in SQL:
SQL
-- Create a table with a JSON column
CREATE TABLE Products (
id INT PRIMARY KEY,
name VARCHAR(50),
price DECIMAL(10,2),
details JSON
);
-- Insert some data into the table
INSERT INTO Products VALUES
(1, 'Laptop', 999.99, '{"brand": "Dell", "model": "XPS 13", "specs": {"processor": "Intel Core i7", "memory": "16 GB", "storage": "512 GB SSD"}}'),
(2, 'Smartphone', 499.99, '{"brand": "Samsung", "model": "Galaxy S21", "specs": {"processor": "Exynos 2100", "memory": "8 GB", "storage": "128 GB"}}'),
(3, 'Tablet', 299.99, '{"brand": "Apple", "model": "iPad Air", "specs": {"processor": "A14 Bionic", "memory": "4 GB", "storage": "64 GB"}}');-- Select the name and brand of the products
SELECT name, JSON_VALUE(details, '$.brand') AS brand
FROM Products;-- Output:
-- name brand
-- Laptop Dell
-- Smartphone Samsung
-- Tablet Apple-- Select the name and specs of the products
SELECT name, JSON_QUERY(details, '$.specs') AS specs
FROM Products;-- Output:
-- name specs
-- Laptop {"processor": "Intel Core i7", "memory": "16 GB", "storage": "512 GB SSD"}
-- Smartphone {"processor": "Exynos 2100", "memory": "8 GB", "storage": "128 GB"}
-- Tablet {"processor": "A14 Bionic", "memory": "4 GB", "storage": "64 GB"}-- Update the price of the laptop by 10%
UPDATE Products
SET price = price * 1.1,
details = JSON_MODIFY(details, '$.price', price)
WHERE id = 1;-- Check the updated data
SELECT *
FROM Products;-- Output:
-- id name price details
-- 1 Laptop 1099.99 {"brand": "Dell", "model": "XPS 13", "specs": {"processor": "Intel Core i7", "memory": "16 GB", "storage": "512 GB SSD"}, "price": 1099.99}
-- 2 Smartphone 499.99 {"brand": "Samsung", "model": "Galaxy S21", "specs": {"processor": "Exynos 2100", "memory": "8 GB", "storage": "128 GB"}}
-- 3 Tablet 299.99 {"brand": "Apple", "model": "iPad Air", "specs": {"processor": "A14 Bionic", "memory": "4 GB", "storage": "64 GB"}}-- Find the products that have more than 8 GB of memory
SELECT name, JSON_VALUE(details, '$.specs.memory') AS memory
FROM Products
WHERE JSON_CONTAINS(details, '"16 GB"', '$.specs.memory');-- Output:
-- name memory
-- Laptop 16 GB
For more information and examples on JSON functions in SQL, you can refer to this article.
Geospatial Functions:
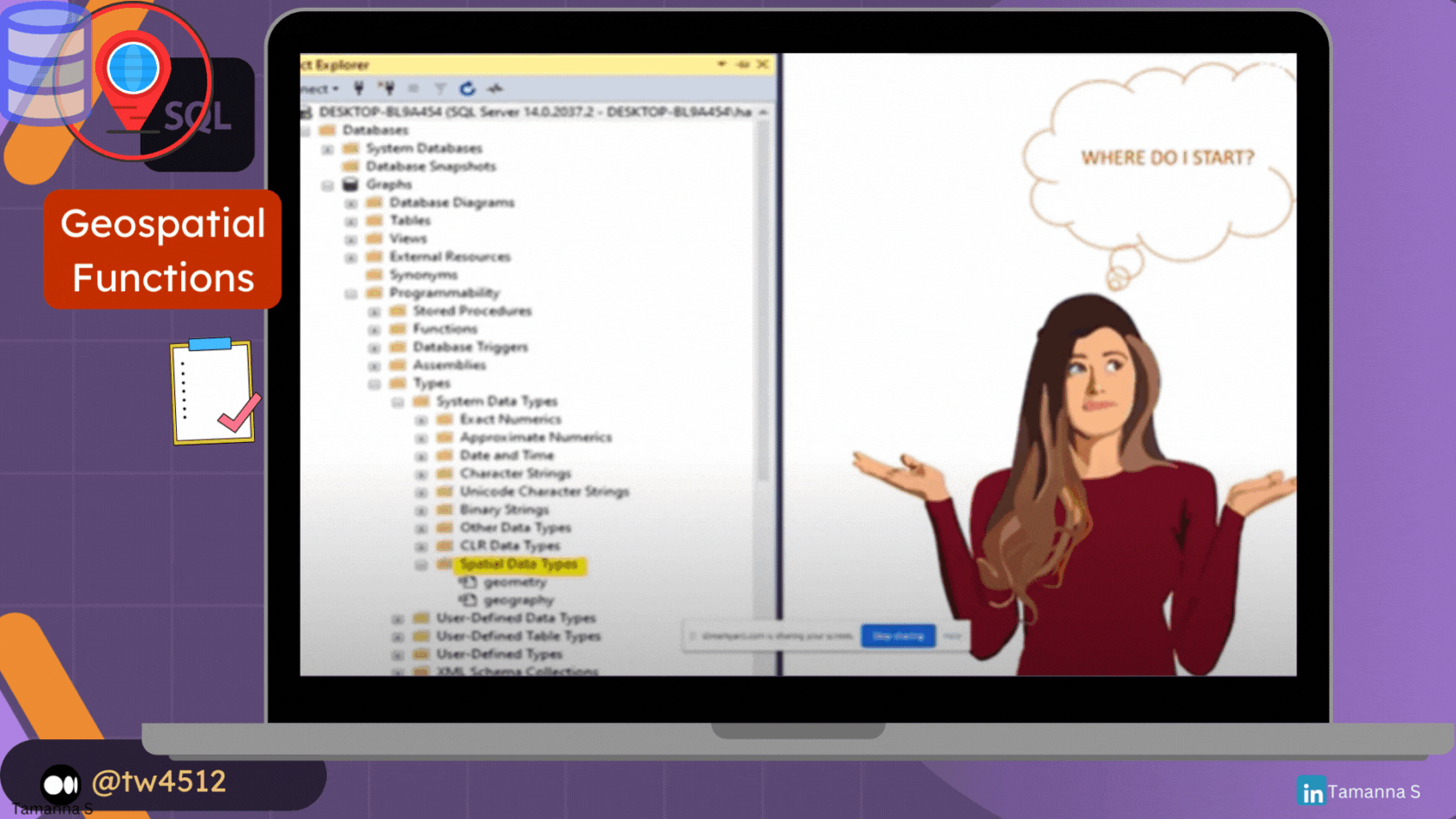
Geospatial data is data that represents the location and shape of geographic features, such as points, lines, polygons, and so on. Geospatial data can be used for various applications, such as mapping, navigation, routing, geocoding, spatial analysis, and more.
SQL also supports geospatial data, and provides several functions to work with geospatial data. For example, you can use the ST_GeomFromText
function to create a geometry object from a text representation, or the ST_AsText
function to convert a geometry object to a text representation. You can also use the ST_Distance
function to calculate the distance between two geometry objects, or the ST_Contains
function to check if one geometry object contains another geometry object. Here is an example of using geospatial functions in SQL:
SQL
-- Create a table with a geometry column
CREATE TABLE Places (
id INT PRIMARY KEY,
name VARCHAR(50),
location GEOMETRY
);
-- Insert some data into the table
INSERT INTO Places VALUES
(1, 'Eiffel Tower', ST_GeomFromText('POINT(2.2945 48.8584)')),
(2, 'Louvre Museum', ST_GeomFromText('POINT(2.3364 48.8606)')),
(3, 'Arc de Triomphe', ST_GeomFromText('POINT(2.2950 48.8738)')),
(4, 'Notre Dame Cathedral', ST_GeomFromText('POINT(2.3499 48.8529)'));-- Select the name and location of the places
SELECT name, ST_AsText(location) AS location
FROM Places;-- Output:
-- name location
-- Eiffel Tower POINT(2.2945 48.8584)
-- Louvre Museum POINT(2.3364 48.8606)
-- Arc de Triomphe POINT(2.295 48.8738)
-- Notre Dame Cathedral POINT(2.3499 48.8529)-- Calculate the distance between Eiffel Tower and Louvre Museum in meters
SELECT ST_Distance(
ST_Transform(ST_GeomFromText('POINT(2.2945 48.8584)', 4326), 3857),
ST_Transform(ST_GeomFromText('POINT(2.3364 48.8606)', 4326), 3857)
) AS distance;-- Output:
-- distance
-- 2953.72017306376-- Find the places that are within 2 km from Eiffel Tower
SELECT name, ST_Distance(
ST_Transform(ST_GeomFromText('POINT(2.2945 48.8584)', 4326), 3857),
ST_Transform(location, 3857)
) AS distance
FROM Places
WHERE ST_Contains(
ST_Buffer(ST_Transform(ST_GeomFromText('POINT(2.2945 48.8584)', 4326), 3857), 2000),
ST_Transform(location, 3857)
);-- Output:
-- name distance
-- Eiffel Tower 0
-- Arc de Triomphe 1679.72017306376
For more information and examples on geospatial functions in SQL, you can refer to this article.
Regular Expressions:
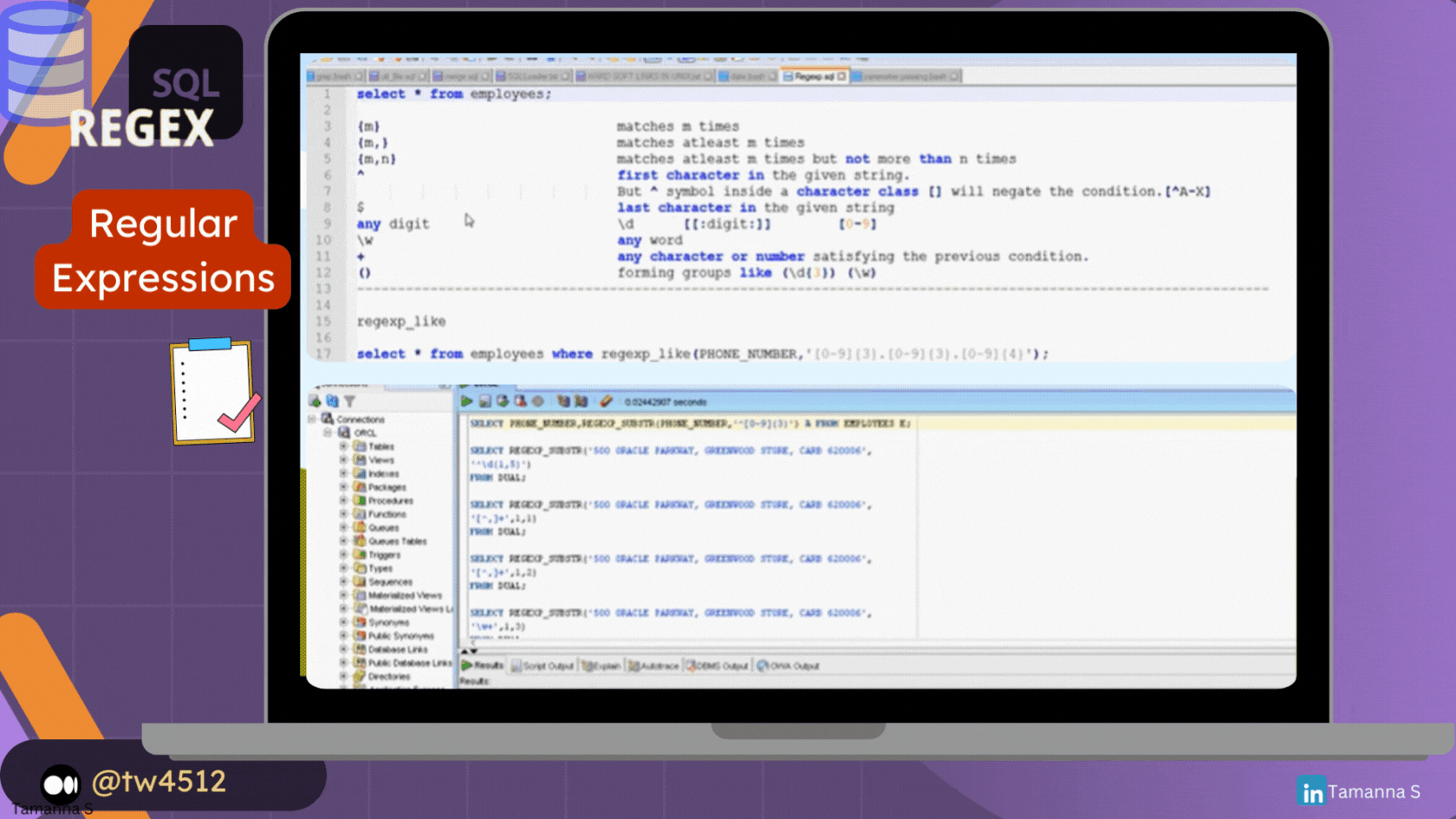
Regular expressions are patterns that can be used to match, search, replace, or validate strings. Regular expressions can be very useful for manipulating and analyzing text data, such as emails, phone numbers, URLs, passwords, and so on.
SQL also supports regular expressions and provides several functions to work with regular expressions. For example, you can use the REGEXP_LIKE
function to check if a string matches a regular expression, or the REGEXP_REPLACE
function to replace a substring that matches a regular expression with another string. You can also use the REGEXP_SUBSTR
function to extract a substring that matches a regular expression from a string, or the REGEXP_INSTR
function to find the position of a substring that matches a regular expression in a string. Here is an example of using regular expressions in SQL:
SQL
-- Create a table with a text column
CREATE TABLE Messages (
id INT PRIMARY KEY,
text VARCHAR(100)
);
-- Insert some data into the table
INSERT INTO Messages VALUES
(1, 'Hello, this is John. My email is john@gmail.com.'),
(2, 'Hi, this is Mary. My phone number is +1-234-567-8901.'),
(3, 'Greetings, this is Bob. My website is https://bob.com
NoSQL vs. SQL:
NoSQL (Not only SQL) is a term that refers to a variety of data models and databases that do not follow the relational model and the SQL language. NoSQL databases are often designed to handle large volumes of unstructured or semi-structured data, such as documents, graphs, key-value pairs, and so on. NoSQL databases are also known for their scalability, performance, and flexibility.
SQL (Structured Query Language) is a standard language that is used to interact with relational databases, which store data in tables with predefined columns and rows. SQL databases are often used for transactional and analytical applications, where data consistency, integrity, and accuracy are important. SQL databases are also known for their reliability, security, and compatibility.
There is no definitive answer to which type of database is better, as it depends on the use case, the data characteristics, and the requirements of the application. However, here is a general comparison of some of the of NoSQL and SQL databases.
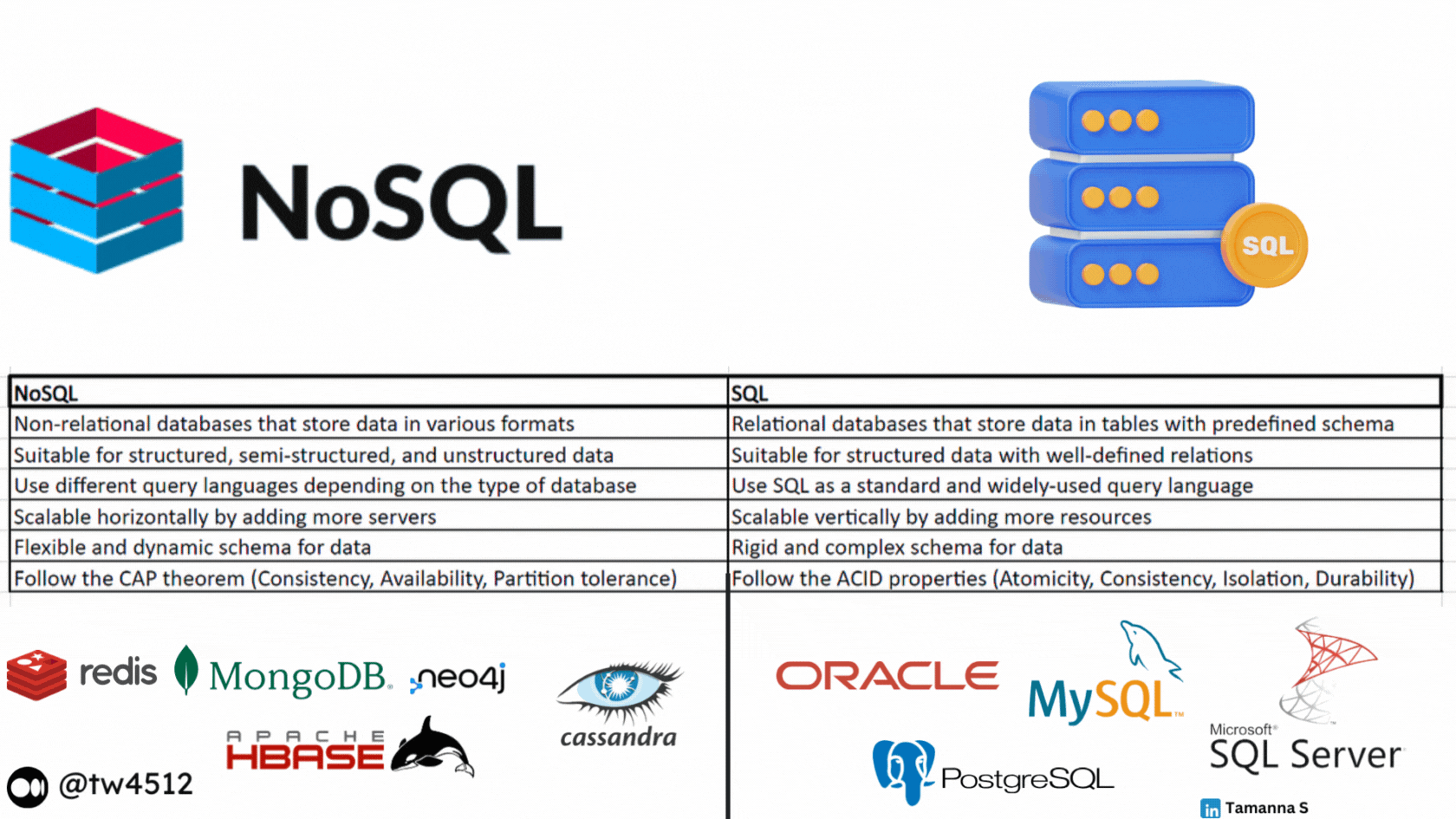
Conclusion:

In this post, we have learned about some of the advanced topics in SQL, such as JSON functions, geospatial functions, regular expressions, and NoSQL vs. SQL. We have also seen how to use these features and functions in SQL queries and examples. These topics can help you enhance your SQL skills and knowledge, and enable you to perform more complex and diverse tasks and queries with SQL.
I hope you enjoyed this post and learned something new and useful. If you did, like π, share, and clap π this blog post, and follow me on Medium and LinkedIn. Also, don’t forget to check out the SQL Learning Series, a comprehensive guide to learning SQL from basic to advanced level.
If you want to learn more about SQL and practice your skills, you can check out [Learn SQL], a platform that provides interactive and engaging courses, quizzes, and exercises on SQL. Learn SQL at your own pace and level, and master the most in-demand data skills today!
1.LearnSQL(Advanced SQL)
2.Advanced SQL π»π
3.Advanced SQL book��π
4.SQL for Students or Beginner’s eππ»π
Thank you for reading and happy learning! π
“SQL, SQL on the wall, who’s the fairest database of them all?” — Anonymous
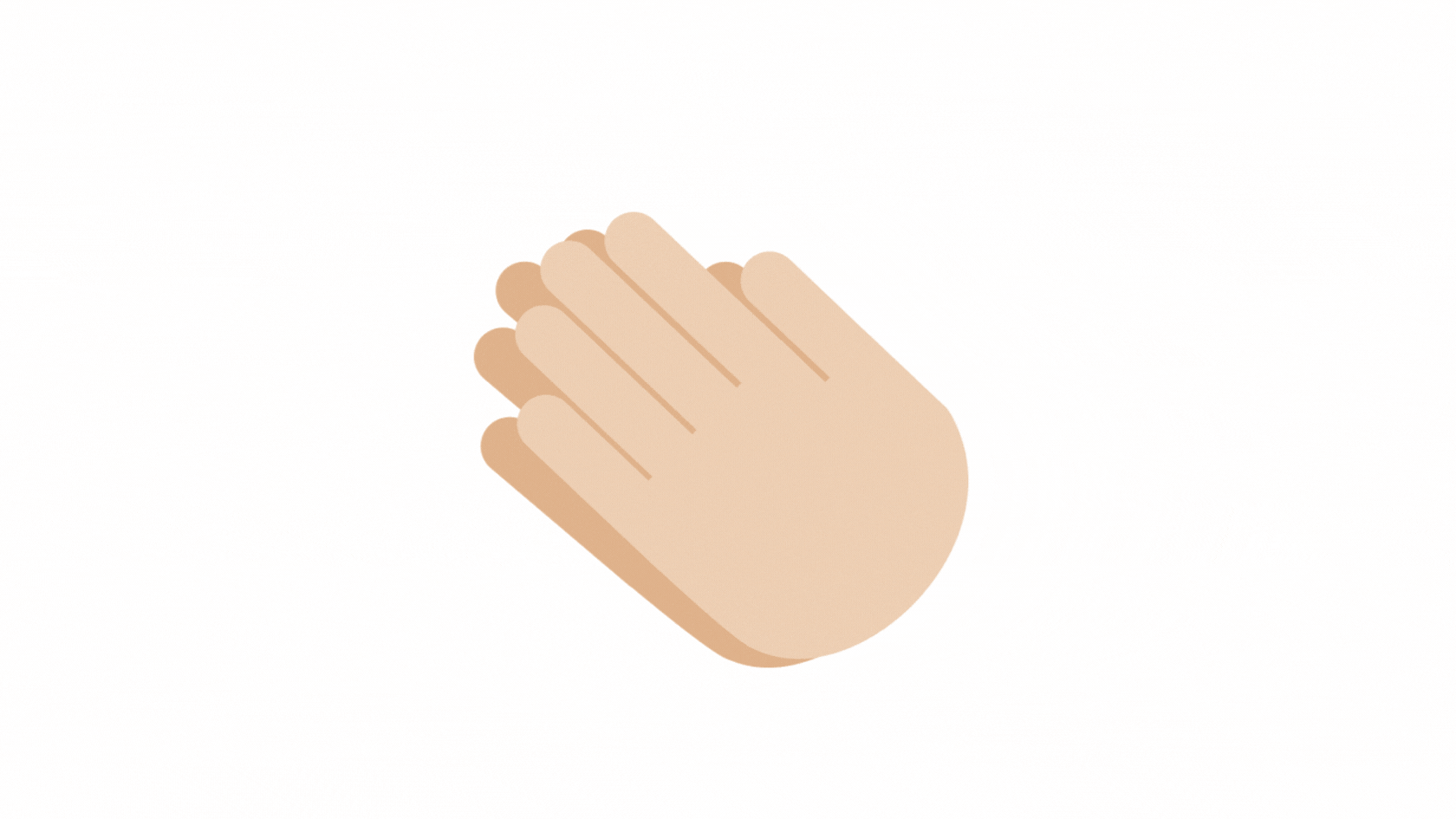
Comments
Post a Comment