MySQL for Beginners: Understanding Common Table Expressions (CTEs).(Part-13)
A Simple Guide to a Powerful SQL Feature
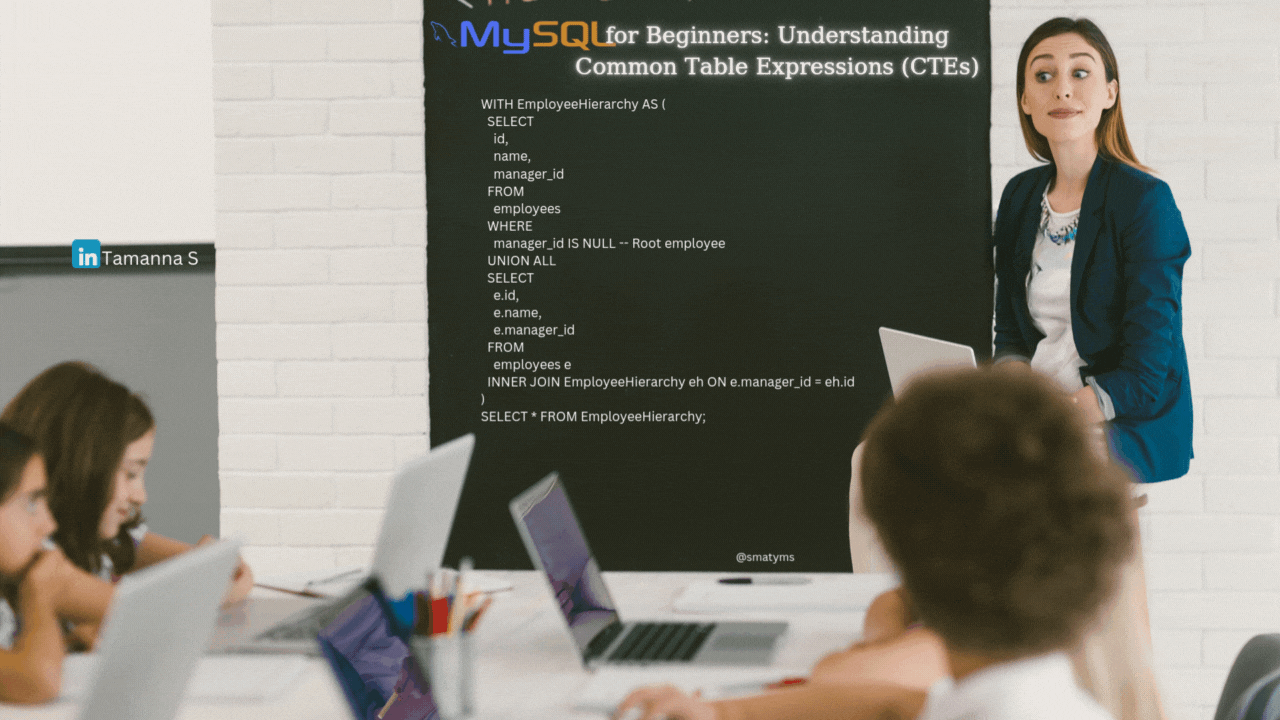
Welcome back to the latest part in our series on MySQL for Beginners! Today, we’ll explore Common Table Expressions (CTEs), an excellent SQL tool that may greatly streamline complicated queries. CTEs are a useful tool to have in your toolbox if you’ve ever had to write complex SQL queries.
Common Table Expressions: What Are They?
Consider yourself constructing a Lego palace. Starting with basic bricks, you can build walls, towers, and eventually a magnificent castle by combining them. CTEs work similarly. They allow you to break down complex queries into smaller, more manageable parts, just like building blocks.
Why Use CTEs?
- Improved Readability: By breaking down complex queries, CTEs make your SQL code easier to understand and maintain.
- Reusability: You can reference a CTE multiple times within a single query, reducing redundancy and improving efficiency.
- Hierarchical Queries: CTEs are particularly useful for handling hierarchical data, such as organizational structures or file systems.
Let’s Build Our First CTE
Suppose we have a simple table called employees with columns id, name, and manager_id. We want to find all employees and their managers in a hierarchical structure. Here’s how we can do it using a CTE:
SQL
WITH EmployeeHierarchy AS (
SELECT
id,
name,
manager_id
FROM
employees
WHERE
manager_id IS NULL -- Root employee
UNION ALL
SELECT
e.id,
e.name,
e.manager_id
FROM
employees e
INNER JOIN EmployeeHierarchy eh ON e.manager_id = eh.id
)
SELECT * FROM EmployeeHierarchy;
Breaking Down the CTE:
- Recursive Part: The first part of the CTE defines the anchor member, which identifies the root employee.
- Recursive Part: The second part recursively joins the employees table with the CTE itself to find the hierarchy.
Visualizing the Hierarchy
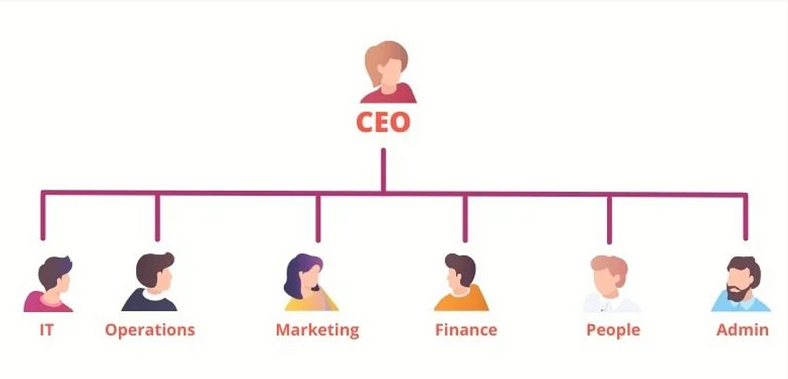
hierarchical tree diagram showing the employee hierarchy
Recursive CTEs: Going Deeper
Recursive CTEs allow you to traverse hierarchical data structures. For example, you can use them to calculate the total sales for each product category, including subcategories.
SQL
WITH RecursiveSales AS (
SELECT
product_id,
product_name,
parent_category_id,
sales_amount
FROM
products
WHERE
parent_category_id IS NULL -- Root categories
UNION ALL
SELECT
p.product_id,
p.product_name,
p.parent_category_id,
p.sales_amount
FROM
products p
INNER JOIN RecursiveSales rs ON p.parent_category_id = rs.product_id
)
SELECT
product_name,
SUM(sales_amount) AS total_sales
FROM
RecursiveSales
GROUP BY
product_name;
Conclusion :
Common Table Expressions are a powerful tool for writing complex SQL queries. By breaking down queries into smaller, more manageable parts, CTEs improve readability, reusability, and performance.
In this blog post, we’ve explored the basics of CTEs and how to use them for both simple and complex queries.
Keep Learning and Keep Growing!
If you found this post helpful, please give it a 👏👏 and follow me on LinkedIn for more SQL tips and tricks. Remember, the journey to SQL mastery is a continuous one, so keep practicing and experimenting!
“The more you know, the more you realize you don’t know.” — Socrates
Comments
Post a Comment